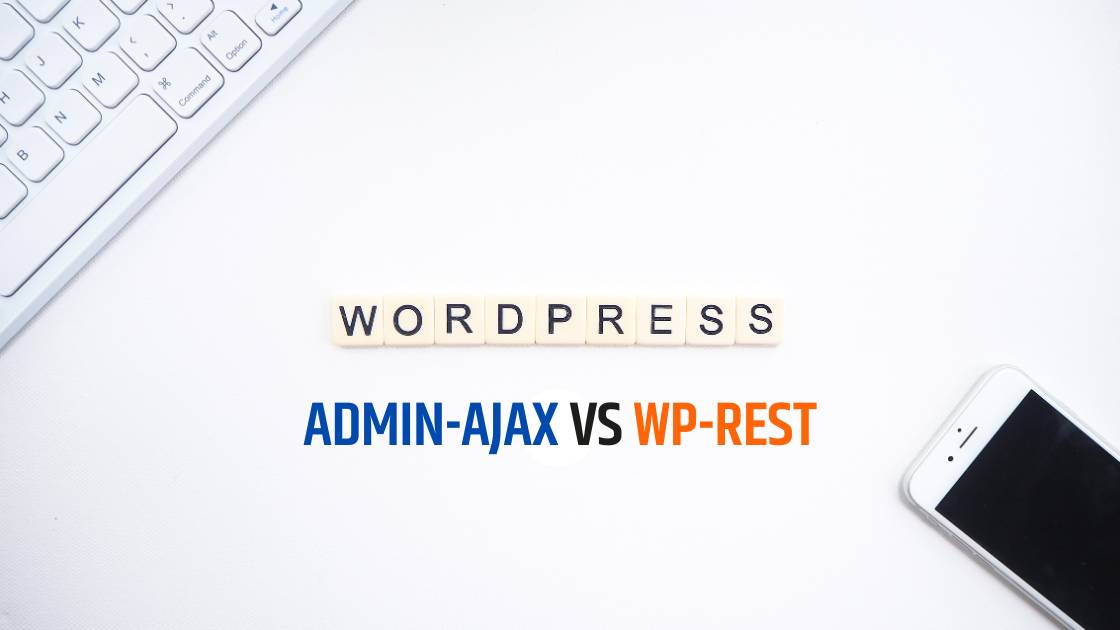
When developing WordPress websites, especially those involving dynamic and interactive elements, developers often rely on asynchronous JavaScript and XML (AJAX) to fetch and send data without reloading the page. WordPress offers two primary methods for handling AJAX requests: the traditional admin-ajax.php
and the more modern WP-REST API. Understanding the differences between these two approaches and their performance implications can help you choose the right tool for your project.
What is Admin-Ajax?
Admin-Ajax is the original method WordPress provided for handling AJAX requests. It involves sending requests to the admin-ajax.php
file, which processes the request and returns the response. This method has been widely used for years and is still supported in WordPress today.
How it Works:
- A JavaScript AJAX call is made to
admin-ajax.php
. - The request is routed through WordPress, loading the full core, plugins, and themes.
- A specified action hook processes the request.
- The response is sent back to the client.
Example Code:
JavaScript:
jQuery.ajax({
url: ajaxurl,
type: 'POST',
data: {
action: 'my_custom_action',
data: 'some_data'
},
success: function(response) {
console.log(response);
}
});
PHP:
add_action('wp_ajax_my_custom_action', 'my_custom_action_function');
add_action('wp_ajax_nopriv_my_custom_action', 'my_custom_action_function');
function my_custom_action_function() {
// Process request and send response
echo 'Response from server';
wp_die(); // Required to terminate immediately and return a proper response
}
What is WP-REST API?
The WP-REST API is a more recent addition to WordPress, providing a standardized way to interact with WordPress data using RESTful principles. It enables developers to create, read, update, and delete content using HTTP requests.
How it Works:
- A JavaScript AJAX call is made to a REST endpoint.
- The request is processed by the designated REST controller.
- The response is sent back to the client.
Example Code:
JavaScript:
fetch('/wp-json/myplugin/v1/myendpoint', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'X-WP-Nonce': wpApiSettings.nonce // Ensure to pass a nonce for security
},
body: JSON.stringify({ data: 'some_data' })
})
.then(response => response.json())
.then(data => console.log(data));
PHP:
add_action('rest_api_init', function() {
register_rest_route('myplugin/v1', '/myendpoint', array(
'methods' => 'POST',
'callback' => 'my_custom_rest_function',
'permission_callback' => '__return_true' // Adjust permission as needed
));
});
function my_custom_rest_function(WP_REST_Request $request) {
$data = $request->get_param('data');
return new WP_REST_Response('Response from server', 200);
}
Performance Comparison
When comparing the performance of admin-ajax.php
and the WP-REST API, several factors come into play:
Initialization Overhead:
- Admin-Ajax: Each request to
admin-ajax.php
initializes the entire WordPress environment, including all active plugins and themes. This can result in significant overhead, especially on large sites. - WP-REST API: REST API endpoints can be more lightweight. While the WordPress environment is still loaded, REST controllers can be optimized to reduce unnecessary processing.
- Admin-Ajax: Each request to
Routing Efficiency:
- Admin-Ajax: Requests to
admin-ajax.php
are routed through WordPress’s core, which can be slower due to the generic handling of AJAX actions. - WP-REST API: The WP-REST API uses a more efficient routing mechanism designed for performance, allowing for faster request handling.
- Admin-Ajax: Requests to
Flexibility and Scalability:
- Admin-Ajax: While functional,
admin-ajax.php
is less flexible and can become cumbersome with complex interactions. It’s less scalable for modern web applications requiring extensive API interactions. - WP-REST API: The WP-REST API is built for flexibility, supporting a wide range of HTTP methods and complex data interactions. It’s more suitable for scalable applications and integrations.
- Admin-Ajax: While functional,
Security:
- Admin-Ajax: Relies on nonce validation for security, but the broader WordPress environment must be loaded to process requests.
- WP-REST API: Also relies on nonce validation but offers more granular control over permissions and security at the endpoint level.
Which One to Choose?
Use Admin-Ajax if:
- You are working on a simple site with limited AJAX interactions.
- You need to maintain compatibility with older plugins or themes.
- Your project relies on established workflows using
admin-ajax.php
.
Use WP-REST API if:
- You are building a modern web application with extensive API interactions.
- You need better performance and efficiency for handling AJAX requests.
- You require more flexibility and scalability for your project.
- You want to implement RESTful principles for better API design.
While admin-ajax.php
has been a reliable method for handling AJAX requests in WordPress, the WP-REST API offers significant performance improvements and flexibility. By reducing initialization overhead and providing efficient routing, the WP-REST API can enhance your site’s responsiveness and scalability, potentially improving performance by up to 10x in some scenarios. For modern WordPress development, especially for dynamic and interactive applications, the WP-REST API is the superior choice.