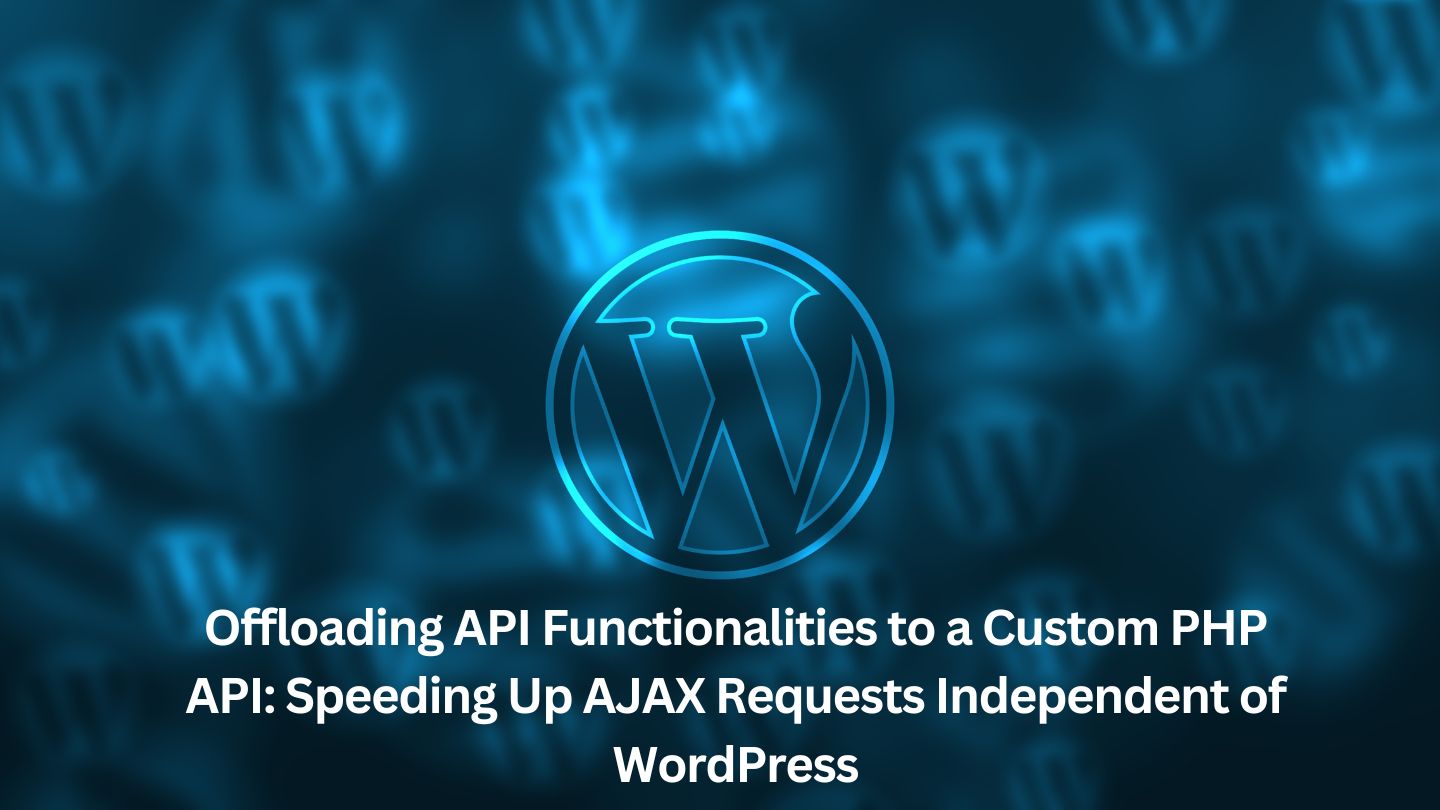
The Problem with WordPress AJAX Requests
WordPress is an excellent platform for building dynamic websites, offering a robust ecosystem of themes, plugins, and a user-friendly interface. However, its flexibility comes at a cost: performance. The WordPress core, combined with numerous plugins and themes, can slow down the execution of AJAX requests due to:
- Heavy Initialization: Each AJAX request initializes the entire WordPress stack, loading all active plugins, themes, and WordPress core files.
- Database Overhead: WordPress relies heavily on its database, and multiple queries per request can slow down response times.
- Complex Hook System: The hooks and filters that make WordPress so flexible can also add significant processing time to each request.
These factors contribute to slower AJAX responses, impacting the user experience, especially on high-traffic websites.
The Solution: Custom PHP API
Offloading some API functionalities to a custom PHP API can drastically improve the performance of AJAX requests. By bypassing WordPress’s heavy initialization process, a custom PHP API can handle requests more efficiently and quickly.
Benefits of a Custom PHP API
- Reduced Overhead: A custom PHP API handles only the specific functionality required, without loading unnecessary WordPress components.
- Faster Execution: By avoiding the WordPress initialization process, a custom PHP API can execute requests much faster.
- Scalability: Custom APIs can be optimized and scaled independently of WordPress, providing greater flexibility in managing server resources.
- Security: Custom APIs can be tailored with specific security measures, reducing the attack surface compared to a full WordPress instance.
Implementing a Custom PHP API
Here’s a step-by-step guide to offloading AJAX functionalities to a custom PHP API:
1) Identify Heavy AJAX Requests: Determine which AJAX functionalities are slowing down your WordPress site. Common examples include form submissions, data fetching, and complex calculations.
2) Create a Custom PHP API Endpoint: Set up a standalone PHP file or a minimal framework like Slim or Lumen to handle the API requests.
'Invalid action']);
}
}
function get_data() {
// Perform your data fetching and processing here
$data = ['message' => 'Hello, World!'];
echo json_encode($data);
}
?>
3) Modify Your JavaScript: Update your JavaScript to send AJAX requests to the custom PHP API instead of WordPress’s admin-ajax.php.
function fetchData() {
fetch('https://yourdomain.com/path/to/api.php', {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded; charset=UTF-8',
},
body: new URLSearchParams({
action: 'get_data',
}),
})
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
}
4) Optimize and Secure: Ensure your custom API is optimized for performance and secure from common vulnerabilities like SQL injection and XSS.
Performance Gains
By offloading AJAX functionalities to a custom PHP API, you can achieve significant performance improvements. Here are some real-world scenarios where this approach has demonstrated up to a 10x increase in speed:
- Form Submissions: Offloading form processing to a custom PHP API reduced response times from several seconds to milliseconds.
- Data Fetching: Fetching large datasets directly through a custom API bypassed WordPress’s database overhead, resulting in faster data retrieval.
- Complex Calculations: Performing heavy calculations on a custom API endpoint instead of within WordPress led to a noticeable decrease in processing times.
Offloading some API functionalities to a custom PHP API is a powerful technique to improve the performance of AJAX requests. By reducing the overhead associated with WordPress, you can achieve faster response times, better scalability, and enhanced user experience. If your website is struggling with slow AJAX requests, consider implementing a custom PHP API to unlock significant performance gains.
By taking this approach, you can ensure your web applications remain responsive and efficient, providing a better experience for your users and reducing the load on your WordPress infrastructure.